Learning Goals
At the end of this Tutorial, you will be able to:
- Assign numbers to variables.
- Perform addition, subtraction, multiplication, and division on numeric variables.
- Use correct operator precedence in arithmetic.
- Use increment and decrement operators on numbers.
Download a new workfile on your computer as described at the link below.
About numeric variables in JavaScript
A numeric variable is a variable that stores a number. A numeric variable can store:
- Integers: The are whole numbers that my be psotive or negative, such as 25 or -10.
- Floating point numbers: Also called floats for short, these are numbers with a decimal point, such as 9.99 or -100.
Assigning numbers to variables
To assign a number to a variable, do not wrap the value in single or double quotes, or backticks.
Copy the following code to your numbers.html workfile.
// ======== ASSIGN NUMBERS TO VARIABLES ========
const myVar1 = 1234; // JavaScript treats myVar1 as a number
const myVar2 = "1234";; // JavaScript treats myVar2 as a string
const myVar3 = '1234'; // JavaScript treats myVar3 as a string
const myVar4 = `1234`; // JavaScript treats myVar4 as a string
console.log(`${myStr1} ${myStr2} ${myStr3} ${myStr4}`); // 1234 1234 1234 1234
Here are two values that JavaScript will accept as valid numbers.
// Valid numbers
let Temperature = -6.3456 // Valid number
let errorRate = .2727 // Valid number
And here are two values that are not valid numbers in JavaScript.
// Not valid numbers - or valid strings either!
let userIncome = 34,000; // Error. Contains a comma
let productID = 645w29; // Error. Contains a letter character
In fact, they are not even valid variable assignments. This is because JavaScript cannot understand what type of data type you want to create. Each line will throw an error.
Delete the above two lines that generate errors from your numbers.html workfile before continuing.
Arithmetic operations
You can perform arithmetic with numeric variables by using the operators set out in the table below.
Operator |
Operator Key Used |
Description |
x + y |
The plus key |
Adds the value of x and the value of y. |
x - y |
The dash key |
Subtracts the value of y from the value of x. |
x * y |
The asterisk key |
Multiplies the value of x by the value of y. |
x / y |
The forward slash key |
Divides the value of x by the value of y. |
See the simple examples below.
// Arithmetic with JavaScript
const firstNum = 12;
const secondNum = 8;
console.log(`Addition (12+8): ${firstNum + secondNum}`); // 20
console.log(`Subtraction (12-8): ${firstNum - secondNum}`); // 4
console.log(`Multiplication (12*8): ${firstNum * secondNum}`); // 96
console.log(`Division (12/8): ${firstNum / secondNum}`); // 1.5
Operator precedence
JavaScript follows the rules of arithmetic when working with numeric variables and numbers:
- Division first, followed by multiplication, then addition and finally subtraction.
For example, the following series of statements gives a result of 11 because JavaScript first multiplies 2 by 3 (resulting in 6) and then adds 5.
// Operator precedence
console.log(`5 + 2 * 3: ${5 + 2 * 3}`); // returns 11
You can force JavaScript to perform calculations in a particular order by using parentheses ().
For example, the following calculation gives a result of 21. JavaScript first adds 5 and 2, because they are within (). And only then multiplies that result by 3 to give 21.
// Operator precedence with parentheses
console.log(`(5 + 2) * 3: ${(5 + 2) * 3}`); // 21
Increment and decrement operators
JavaScript supports increment ++ and decrement -- prefix operators that increase or reduce the value of a numeric variable by one.
See the sample code below.
// Increment and decrement operators
let myNum1 = 7;
let myNum2 = 24;
console.log(`myNum1 is 7`);
console.log(`Increment operator (++myNum1) is ${++myNum1}`); // 8
console.log(`myNum2 is 24`);
console.log(`Decrement operator (--myNum2) is ${--myNum2}`); // 23
Using the +=
operator with numbers
In the Working with strings Tutorial, your learnt how to use compound assignment += operator to work with text. You can also use this operator to work with numeric values.
The += operator:
- Adds the value on the right to the variable on the left
- Assigns the result back to the variable on the left
Here are two examples:
// === Compound assignment operator += with numbers ===
let count = 5;
console.log(count); // 5
count += 3; // count is now 8
count += 2; // count is now 10
// Keeping score in a computer game
let playerScore = 100;
playerScore += 50; // Player hits target
playerScore += 25; // Player collects coin
playerScore += 75; // Player completes level
console.log(playerScore); // 250
// Calculating compound interest
let balance = 1000;
let interest = balance * 0.05; // 5% interest
balance += interest;
console.log(balance); // 1050
In the Practical functions Tutorial, you will learn to use numeric formating and calculations to solve everyday coding problems.
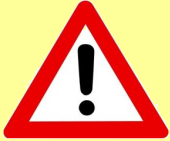
JavaScript has some VERY WEIRD behaviours when dealing with numbers. For this reason, it is recommended to test every variable to ensure it really is a numeric variable before using it in a calculation. Also, JavaScript in some circumstances will convert a string to a number and vice vera. These issues are dealt with in the Testing for weird values Tutorial.
More learning resources
Tutorial Quiz
Tutorial Podcast
Try it yourself
In your workfile...
---
Write code to calculate the total cost of a shopping cart where each item costs $9.99 and a user buys 3 items.
---
Create a temperature converter that takes a Celsius temperature and converts it to Fahrenheit using this formula: (C × 9/5) + 32.
Sample AI prompts
Explain why 5 + 2 * 3
equals 11 but (5 + 2) * 3
equals 21. What JavaScript rule determines this?
What's the difference between these two variable declarations and why does it matter?
let num1 = 42;
let num2 = "42";