Learning Goals
At the end of this Tutorial, you will be able to:
- Declare string variables in JavaScript and use typeof to test that a variable contains a string.
- Use various string methods to investigate and transform string variables.
- Concatenate (join) strings and use the compound assignment += operator.
Download a new workfile on your computer as described at the link below.
About string variables in JavaScript
What is usually referred to as ‘text’ in everyday language in programming is called a string. A string is simply a sequence of one or more characters – letters, numbers, blank spaces or punctuation symbols.
Assigning string content to variables
To assign string content to a variable, wrap the content in single or double quotes. Copy the sample code below into your strings.html workfile.
// ======== ASSIGN CONTENT AS A STRING ========
const myStr1 = "Hello"; // JavaScript treats myStr1 as a string
const myStr2 = 'World'; // JavaScript treats myStr2 as a string
const myStr3 = " "; // JavaScript treats myStr3 as a string
console.log(`${myStr1} ${myStr2} ${myStr3}`); // Hello World
Note that a whitespace character is treated as a valid string. The myStr3 variable is not empty.
With the let keywords, an empty string can be declared (created and named) in one statement and then initialised (first value assigned to it) in another.
// Declare and initialise a string variable
let myStr4 = ""; // Creates an empty string variable
console.log(myStr4); // Outputs an empty string
myStr4 = "Some text here"; // String now initialised with content
A string declared with let (but not const) can be reassigned.
// Re-assign content in string variable
let myStr5 = "Things are looking good.";
myStr5 = "Things are looking even better."; // New content assigned
console.log(myStr5); // Outputs new re-assigned content
Attempting to reassign a string variable created with const throws an error in the Console as shown below.
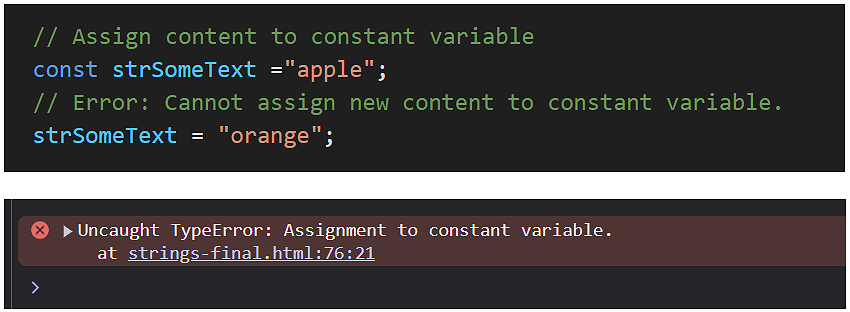
The typeof
operator
If you are unsure about a variable’s data type, you can use the typeof operator on the variable as shown below.
// All statements return 'string'
console.log(`Data type of myStr1: ${typeof myStr1}`);
console.log(`Data type of myStr2: ${typeof myStr2}`);
console.log(`Data type of myStr3: ${typeof myStr3}`);
console.log(`Data type of myStr4: ${typeof myStr4}`);
console.log(`Data type of myStr5: ${typeof myStr5}`);
About character positions in a string
JavaScript assigns an index number to each character in a string, starting with 0. This is called zero-based indexing. Each character in a string can be accessed by its position-based index number within square brackets [n]. See below.
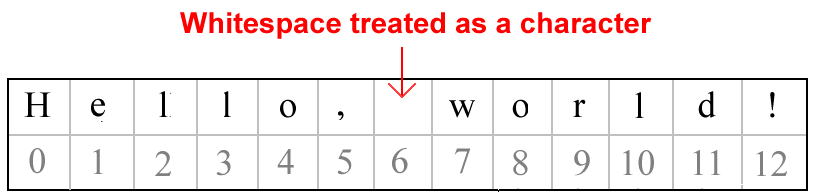
myStr6[0] |
Outputs the first character in the string |
myStr6[5] |
Outputs the sixth character in the string |
myStr6[12] |
Outputs the last character in the string |
Copy the sample code below into your strings.html workfile.
// Using [] notation to access position of characters within string
let myStr6 = "Hello, World!";
console.log(`Position [0]: ${myStr6[0]};`) // H
console.log(`Position [5]: ${myStr6[5]};`) // ,
console.log(`Position [6]: ${myStr6[6]};`) // whitespace
console.log(`Position [7]: ${myStr6[7]};`) // W
console.log(`Position [12]: ${myStr6[12]};`) // !
The first character in the string is H corresponds to index position 0. The last character is !, which corresponds to index position 12.
Note that the whitespace character, entered with the Spacebar also has an index value, at 6.
Being able to access every character in a string with the square bracket [] notation makes it easy to access and manipulate strings.
Finding the length of a string
Every string has a .length property that reveals the number of characters in the string. The syntax is as follows.

Copy the following example to your strings.html workfile. It should output a string length of 13 to the console.
// ======== CHECKING STRING CONTENT ========
// String length
console.log(myStr6.length); // 13
Note: the length of a string is always one number greater than the string’s highest index number.
Checking string content with methods
In JavaScript, the word method describes some action you can perform on a item of code such as a variable. Note that:
- A method ends with a parenthesis ().
- Some methods take arguments (values) inside the parenthesis.
Below is a table of some common string methods.
Method |
Description |
.includes() |
Checks if a string contains a specified character(s). |
.startsWith() |
Checks if a string starts with a specified character(s). |
.endsWith() |
Checks if a string ends with a specified character(s). |
.indexOf() |
Outputs the position of the first occurrence of a specified character(s) in a string. |
Here are some examples of using such methods to investigate the content of a string variable.
// Testing for character(s) present in string
console.log(myStr6.includes("World")); // boolean true
console.log(myStr6.startsWith("Hello")); // boolean true
console.log(myStr6.endsWith("!")); // boolean true
// Finding position of character(s) in string
console.log(myStr6.indexOf("World")); // 7
console.log(myStr6.indexOf("cheese")); // -1 (Not found in string)
Transforming string content
JavaScript also offers methods for reformatting or changing the content of a string variable. Copy the following examples to your strings.html workfile.
// ======== TRANSFORMING STRING CONTENT ========
let strUserName = " John Smith ";
// Remove whitespace
console.log(strUserName.trim()); // "John Smith" (without quotes)
// Change case
console.log(strUserName.toLowerCase()); // " john smith " (without quotes)
console.log(strUserName.toUpperCase()); // " JOHN SMITH " (without quotes)
These methods are useful for cleaning up user input to forms or for formatting text for display.
In addition to whitespaces, .trim() also removes tab, no-break space and line terminator characters.
Note that .trim() only works for characters at the start and end of a string. Any spaces between words are unaffected.
Chaining string methods
You can chain (combine) string methods together in a single expression. See below.
// Chaining methods
console.log(strUserName.trim().toLowerCase());
Extracting a substring from a string (slicing)
Sometimes, you will want to extract and work with part of a string or substring. You can use the .slice() method for this task. Copy the examples below.
// Extracting substrings from a string
const strForSplicing = "JavaScript is awesome";
console.log(strForSplicing.slice(0, 10)); // JavaScript
console.log(strForSplicing.slice(11)); // is awesome
console.log(strForSplicing.slice(-7)); // awesome
.slice(start,end) |
Outputs a new string extracted at the specified start position from the original string. If the optional end argument is omitted, the new string will include every character of the original string from the start up to the end. When you use a negative number as the only argument, this method counts backwards from the end of the string. |
Replacing content in a string
JavaScript provides two methods that will search a string for a value and replace it with a new value. Copy the examples below to your strings.html workfile.
// ======== REPLACING STRING CONTENT ========
const txtMessage = "Hello World";
// Replace first occurrence
console.log(txtMessage.replace("o", "0")); // Hell0 World
// Replace all occurrences
console.log(txtMessage.replaceAll("o", "0")); // Hell0 W0rld
Both these methods are case-sensitive.
String methods do not change string values
Important: string methods do not change the original string value. Instead, a string method will create a new string with the changes applied.
To demonstrate this fact, copy the examples below to your strings.html workfile. In every case, you can see that the original string is unchanged.
// === STRING METHODS DO NOT CHANGE ORIGINAL STRING VALUES ===
// Original string
let strMessage = "Hello from JavaScript string variable";
console.log(strMessage); // Original: Hello from JavaScript string variable
// This does NOT change the original string
strMessage.toUpperCase();
console.log(strMessage); // Unchanged: Hello from JavaScript string variable
Typically, the reason you apply a string method is that you want to change the original value. To do this, you need to reassign the transformed value back to the original string. See below.
// To actually change the value, you need to assign the result back
strMessage = strMessage.toUpperCase();
console.log(strMessage); // HELLO WORLD
Here's another example with multiple string operations
let sampleText = " JavaScript ";
// These methods don't modify the original string
sampleText.trim();
sampleText.replace("J", "j");
console.log(sampleText); // " JavaScript " (without quotes)
// To make actual changes, assign the output back
sampleText = sampleText.trim().replace("J", "j");
console.log(sampleText); // "javaScript" (without quotes)
In summary, if you want to modify a string value, you need to:
- Use the string method to create the new string
- Assign that new string back to a variable (either the original variable or a new one)
Concatenating (joining) strings together
Joining two or more string variables is known as concatenation. You can achieve this using template literals. Copy the example below.
// ======== CONCATENATING STRINGS ========
// Joining strings with template literals
let txtFirstName = "John";
let txtLastName = "Lennon";
let txtFullName = `${txtFirstName} ${txtLastName}`;
console.log(`Full name: ${txtFullName}`); // John Lennon
A second and more common option for joining strings is to use the + operator.
// Joining strings with the + operator
txtFullName = txtFirstName+ " " +txtLastName;
console.log(`Full name: ${txtFullName}`); // John Lennon
Both methods will output the same result.
Using the +=
operator with strings
JavaScript offers a compound assignment += that combines two operations:
- It adds the value on the right to the variable on the left
- It assigns the result back to the variable on the left
Here are two examples:
// Compound assignment operator +=
let greeting = "Hello";
greeting += " there"; // Hello there
greeting += "!"; // Hello there!
console.log(greeting);
let story = "Once upon a time";
story += " there was a programmer";
story += " who loved to code";
story += " and debug all day long.";
console.log(story)
However, in certain circumstances, the + and += operators can create unexpected results. See the Testing for weird values Tutorial.
In the Practical functions Tutorial, you will learn to apply string methods to solve everyday coding problems.
Try it yourself
In your workfile...
---
Create three variables with your first name, last name, and favorite color. Use a template literal to output: "Hi! My name is [firstName] [lastName] and my favorite color is [color]."
---
Create a variable with the text: "JavaScript is fun!"
- Output its total length
- Output the first character using [] notation
- Output the last character using [] notation
- Output the start position of the word "fun" using [] notation
---
Start with the text: "The quick brown fox"
- Extract just the words "quick brown"
- Replace "fox" with "dog"
- Add the text "jumps over the lazy dog" to the end
More learning resources
Tutorial Quiz
Tutorial Podcast
Sample AI prompts
What is the practical purpose of string methods like .trim()
, .toUpperCase()
, and .toLowerCase()
? Give me very basic real-world scenarios where each would be useful.
Review this code and suggest improvements:
let userName = ' john smith ';
userName = userName.toUpperCase();
let finalName = userName.trim();
console.log(finalName);
Can you explain the difference between using .slice()
and .indexOf()
on strings? Please provide simple examples showing when to use each.