Learning Goals
At the end of this Tutorial, you will be able to:
- Understand how spreadsheet data maps to arrays of objects
- Display structured data in the console and in HTML
Download the exercise file on your computer as described at the link below.
Arrays and objects: a review
In the previous Introduction to objects and Introduction to arrays Tutorials, you learnt the following:
- Object (Lots of data about one thing): A collection of properties with two parts: a key (such as firstName) and a value (such as Luis).
Each property value can be accessed with the object name, a dot ., and the key name (such as objUser.firstName).
- Array (Lots of things): An ordered list of items of the same data type, such as strings or numbers. A comma , character separates the items in the list.
Each array item can be accessed by its index position inside square brackets [n], beginning at zero. For example, arrEmployeeIDs[3] would be the fourth item in the array, 1237.
About arrays of objects
Arrays of objects combine the power of arrays with the structure of objects.
Consider the stylesheet-style table of three products below.

In JavaScript, you can represent this table as an array. Each row in the table is an object. And each column is an object property.
Copy this code to your arrays-objects.html workfile:
// Creating an array of objects
const arrProducts = [
{
productID: 1001,
name: "Laptop",
price: 999.99,
inStock: true
},
{
productID: 1002,
name: "Mouse",
price: 24.99,
inStock: true
},
{
productID: 1003,
name: "Keyboard",
price: 59.99,
inStock: false
}
];
console.log(arrProducts);
Arrays of objects are a very common data structure in JavaScript. They are typically used to store collections of related data such as lists of users, product catalogues, social media posts, and transaction records.
Each item in the array is an object that follows the same property structure, making it easy to organise and process data.
Note how:
- The entire array begins and ends with square brackets []
- Each object is surrounded by curly braces {}
- As in regular arrays, the objects are separated by commas ,
The general form of an array of objects is below.
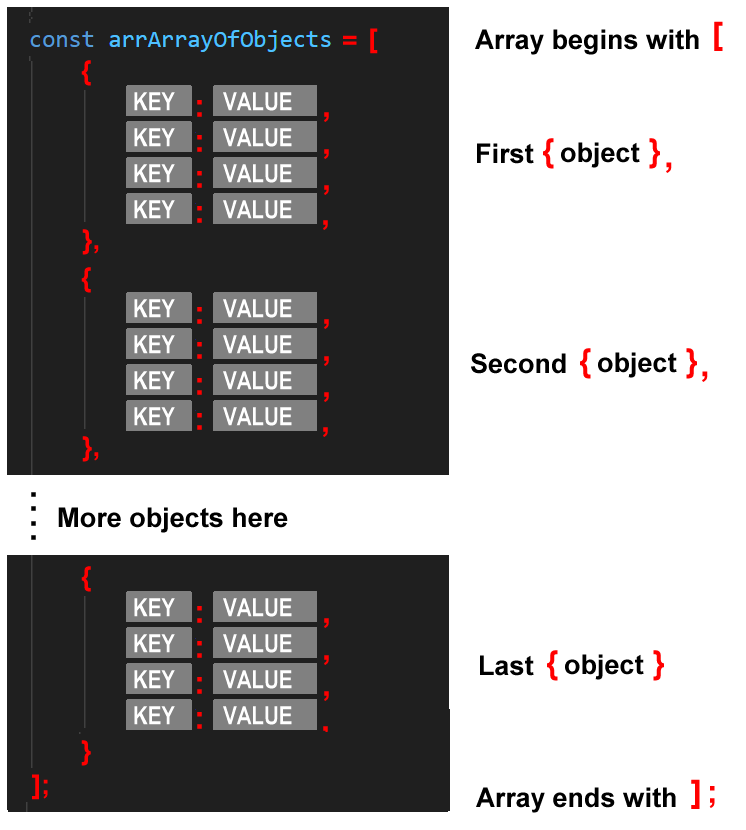
For convenience, the objects in an array are often typed on a single line. Copy the example below.
// Creating an array with objects on a single line
const arrUsers = [
{ firstName: "Alice", age: 25, city: "New York" },
{ firstName: "Bob", age: 30, city: "Los Angeles" },
{ firstName: "Charlie", age: 35, city: "Chicago" }
];
console.log(arrUsers);
Displaying data from an array of objects
A key difference between a single object and objects in an array is that objects in an array do not have a name.
For an individual object, you access a property value by entering the object name (such as objLaptop) and the property key (such as price). See below.
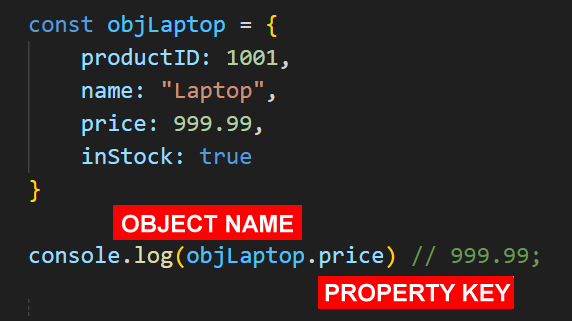
However, the objects in an array don't have individual names. Instead, you access data from a particular object in an array by its index position and its property key.
Copy the code below that displays selected property values from your two arrays of objects.
// Displaying some data from the arrProducts array of objects
console.log(`ID of product 0: ${arrProducts[0].productID}`); // 1001
console.log(`Name of product 1: ${arrProducts[1].name}`); // Mouse
console.log(`Price of product 2: ${arrProducts[2].price}`); // 59.99
// Displaying some data from the arrUsers array of objects
console.log(`First Name of user 0: ${arrUsers[0].firstName}`); // Alice
console.log(`Age of user 1: ${arrUsers[1].age}`); // 30
console.log(`City of user 2: ${arrUsers[2].city}`); // Chicago
Looping through arrays of objects
Arrays may have many hundreds of objects, each with lots of properties. So it is impractical to enter a line of code to display each property value of every object.
The solution is to use a .forEach() loop on each object in the array. Copy the code below.
// Loop through arrProducts and output formatted data
arrProducts.forEach(product => {
console.log("Product Details:");
console.log(`ID: ${product.productID}`);
console.log(`Name: ${product.name}`);
console.log(`Price: ${product.price}`);
console.log(`In Stock: ${product.inStock}`);
console.log("---------------"); // Separator between products
});
Copy this code to output data from the arrUsers array of objects.
// Loop through arrUsers and output formatted data
arrUsers.forEach(user => {
console.log("User Details:");
console.log(`First Name: ${user.firstName}`);
console.log(`Age: ${user.age}`);
console.log(`City: ${user.city}`);
console.log("---------------"); // Separator between users
});
Reformatting the output from an array of objects
When displaying data from an array of objects, you can format the output in a more readable way.
For example, you could reformat the output from arrProducts as shown below.
// Reformat output from arrProducts
arrProducts.forEach(product => {
console.log(`Product: ${product.name.toUpperCase()} (ID: ${product.productID}) €${product.price}`);
// Variable to hold stock status
let stockStatus;
// Determine stock status using if...else
if (product.inStock) {
stockStatus = '✓ Currently Available';
} else {
stockStatus = '✗ Out of Stock';
}
console.log(`In Stock? ${stockStatus}`);
console.log("---------------"); // Separator between products
});
Sometimes, string values in the array of objects may contain extra spaces. For example, add some extra space characters before and after the firstName property in the arrUsers array.
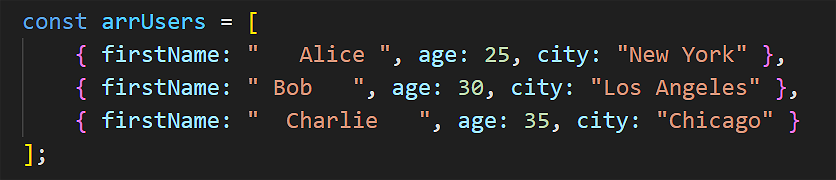
To remove these extra spaces from the output of the arrUsers array, you could do the following.
// Removing extra spaces from formatted output
arrUsers.forEach(user => {
console.log("User Details:");
console.log(`First Name: ${user.firstName.trim()}`);
console.log(`Age: ${user.age}`);
console.log(`City: ${user.city}`);
console.log("---------------"); // Separator between users
});
Display the data on a web page
As a final task, output the data from the two arrays of objects in the HTML of a web page. Here are the steps:
- In the HTML of the arrays-objects.html workfile, create a new <div> tag with an id of output-products.
- Copy the code below into your workfile.
// Displaying data from arrProducts in HTML // Get reference to the div container to hold the products output const containerProducts = document.getElementById('output-products'); // Loop through each product arrProducts.forEach(product => { // Create a sub-heading tag for each product's name const headingProducts = document.createElement('h3'); // Add product name to sub-heading tag headingProducts.innerText = product.name; // Create paragraph for each product's details const detailsProducts = document.createElement('p'); // Check stock status let stockStatus; if (product.inStock) { stockStatus = 'In Stock'; } else { stockStatus = 'Out of Stock'; } // Add product details to paragraph page detailsProducts.innerText = `Product ID: ${product.productID} Price: €${product.price} Stock Status: ${stockStatus}`; // Append heading and paragraph tags to div container containerProducts.appendChild(headingProducts); containerProducts.appendChild(detailsProducts); });
The HTML output should look similar to that below.
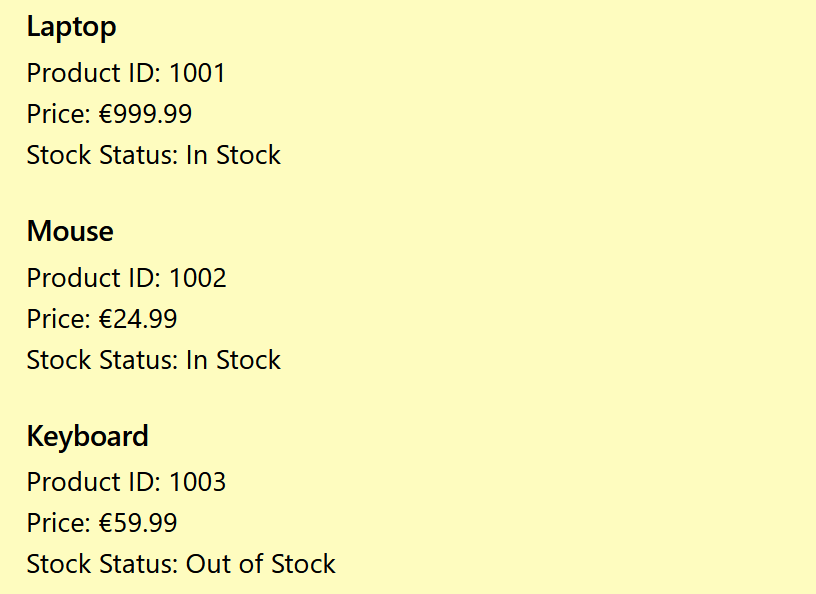
To output the data from the arrUsers array of objects in the web page, follow these steps:
- In the HTML of the arrays-objects.html workfile, create a new <div> tag with an id of output-users.
- Copy the code below into your workfile.
// Displaying data from arrUsers in HTML // Get reference to the div container to hold the users output const containerUsers = document.getElementById('output-users'); // Create a sub-heading tag for entire output const headingUsers = document.createElement('h3'); headingUsers.innerHTML = "<b>List of users</b>"; // Append heading to div container containerUsers.appendChild(headingUsers); // Loop through each user arrUsers.forEach(user => { // Create paragraph tag for user details const detailsUsers = document.createElement('p'); // Add user details to paragraph detailsUsers.innerText = `First Name: ${user.firstName.trim()} | Age: ${user.age} | City: ${user.city}`; // Append paragraph to div container containerUsers.appendChild(detailsUsers); });
The HTML output should look similar to that below.
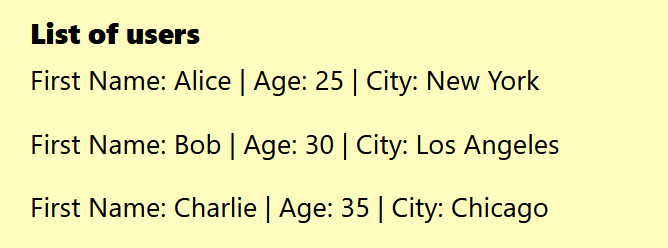
Try it yourself
In your workfile...
---
Create an array called arrBooks
with at least four book objects. Each book object should have these properties:
- title (string)
- author (string)
- year (number)
- isRead (boolean)
You use AI to generate the details.
Using a .forEach()
loop, display each book's information in the console and in HTML.
---
More learning resources
Tutorial Quiz
Tutorial Podcast
Sample AI prompts
I'm learning about arrays of objects in JavaScript. Could you help me understand them better by:
- Creating an example array of objects representing a playlist of songs
- Explaining how each part of the syntax works (brackets, braces, commas, etc.)
- Showing me how to access specific properties like the title of the second song
- Demonstrating how to loop through the array with .forEach() and display the data
- Please explain each step as if you're teaching a beginner.