Learning Goals
At the end of this Tutorial, you will be able to:
- Create and populate an array with square brackets [] notation.
- Use the .length property to find the number of items in an array.
- Use array indexes to access individual items in an array.
- Test for an array with the Array.IsArray() method.
- Loop through the items in an array with the .forEach() method.
- Destructure individual items of an array to variables.
Download a new workfile on your computer as described at the link below.
Storing spreadsheet-type data in arrays
In the previous Introduction to objects Tutorial, you learnt that a JavaScript object can be used to store the related data in a single row of a spreadsheet such as shown below.
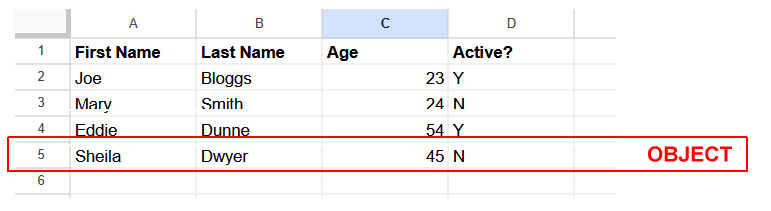
But what about all the rows of a spreadsheet? How can they be stored in JavaScript? The answer is: in an array.
You can think of an array as a list of things. The things (usually called items or elements) in an array could be objects. But they can also be simple variables such as strings or numbers.
In an array, the order in which the items are stored is important. For this reason, an array is more propertly defined as an ordered list of items.
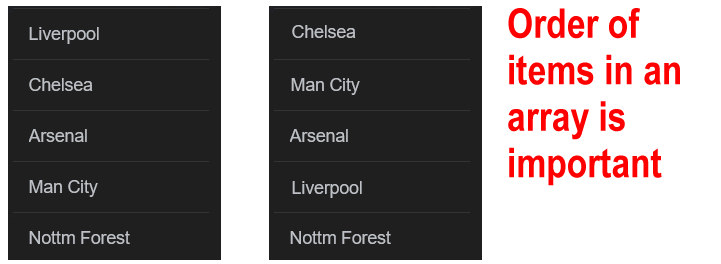
Naming your arrays
Object names follow the usual JavaScript rules: they are case-sensitive and cannot contain spaces or the dash (-) character. Here are two tips:
- Begin array names with arr, such as arrStudents and arrEmployees.
- Use plural nouns for array names, such as arrProducts rather than arrProduct and arrUsers rather than arrUser.
Creating an array with []
notation
You can create an array with an array name and pair of square brackets []. This is called literal notation.
Copy the following array code to your arrays.html workfile.
// Declaring an array with items on separate lines and a trailing comma
const arrUserFirstNames = [
"Camille",
"Emma",
"Gabriel",
"Romy",
];
As you can see above, there is a , character after each item. Also, a ‘trailing comma’ is added after the final item "Romy". Developers sometimes add an optional trailing comma to make it easier to insert/remove items later.
You can see some further examples of arrays below.
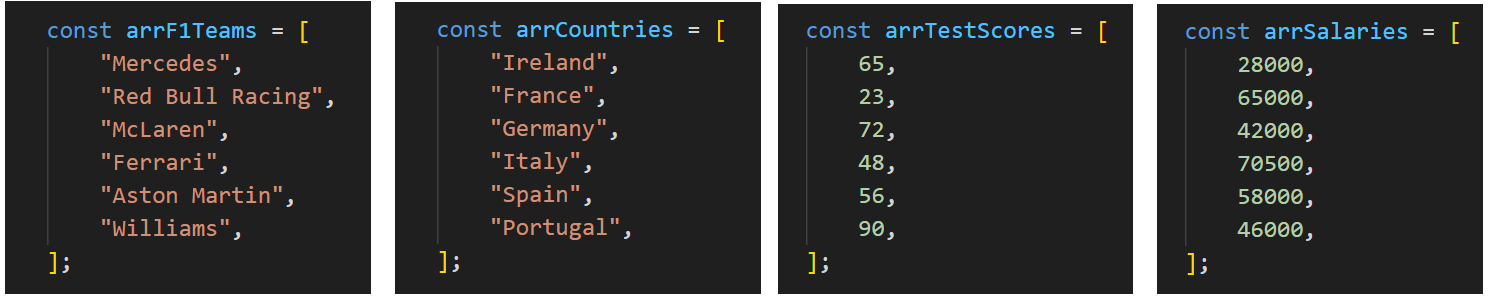
In practice, arrays are commonly typed on a single line. For extra readability, individual array items are separated by a single space.
Copy these three arrays to your workfile.
const arrUserIDs = [4032, 5229, 1234, 6317];
const arrCars = ["Volvo", "Citreon", "BMW", "Opel", "Peugeot", "Ford"];
const arrPrices = [11.99, 24.50, 34.25, 49.99];
Strings are typically wrapped with double "" rather than single quotes ''.
It is better to use const rather than let when creating arrays. This does not prevent your code from modifying items in an array later as needed. But it does prevent the array from being accidentally overwritten by another another array (or variable) with the same name that might exist elsewhere in the same scope.
Here is the general form of an array of numbers.

And here is the general form of an array of strings.

In JavaScript, items in an array need not be the same data type. But it is not recommended to mix multiple data types in the same array.
Arrays and index values
Each array item has an identifying integer (whole number) known as an index. Indexes begin at zero and the number is written inside square brackets [].

For example, an array with four items has the following indexes: [0], [1], [2] and [3]. JavaScript arrays are said to be zero-indexed.
In this respect, items in an array are similar to characters in a string variable.
Add the following code to your arrays.html workfile to output items from the three arrays, as identified by their index position.
// === Outputting array items by index position ===
console.log(`First item of arrUserFirstNames array: ${arrUserFirstNames[0]}`);
console.log(`First item of arrPrices array: ${arrPrices[1]}`);
console.log(`First item of arrCars array: ${arrCars[2]}`);
console.log(`First item of arrUserIDs array: ${arrUserIDs[3]}`);
The .length
property of an array
Every array has a .length property that reveals the number of items in the array. The syntax is as follows.

Here is some sample code to copy.
// === Array length property ===
console.log(`Number of items in arrUserFirstNames: ${arrUserFirstNames.length}`);
// 4
console.log(`Number of items in arrPrices: ${arrPrices.length}`);
// 4
Remember: the length of an array is always one number greater than the array's highest index number.
We can use this to access the last item in an array - the item with the highest index number. See below.
// === Accessing last item in array ===
console.log(`Last item of arrCars array: ${arrCars[arrCars.length-1]}`);
// Ford
console.log(`Last item of arrStudentIDs array: ${arrUserIDs[arrUserIDs.length-1]}`);
// 6317
Arrays and the typeof
operator
Arrays in JavaScript are a special type of object. The typeof operator when applied an array will return object.
This is same value that is returned when typeof is applied to a null variable. See below.
// === Using typeof with a null variable and arrays ===
let someVar = null;
console.log(`Type of someVar: ${typeof someVar}`);
// Outputs object
console.log(`Type of arrUserFirstNames: ${typeof arrUserFirstNames}`);
// Also outputs object
console.log(`Type of arrPrices: ${typeof arrPrices}`);
// Also outputs object
Testing with the Array.IsArray
method
To check that some item in your code is actually an array, you can use the Array.isArray() method. You pass the item name to the method as an argument.
This returns a boolean true if the item is an array and false otherwise.
Test the code below runs correctly.
// === Testing for an array ===
// Check if arrCars is an array
if (Array.isArray(arrCars)) {
console.log("arrCars is an array.");
} else {
console.log("arrCars is not an array.");
}
// Check if someVar is an array
if (Array.isArray(someVar)) {
console.log("someVar is an array.");
} else {
console.log("someVar is not an array.");
}
Looping through an array
Often, you will want to perform the same operation on all the items in an array - such as displaying their values.
In modern JavaScript, the preferred way of looping through an array is to use the forEach() method. See the example below.
// === Looping through array values ===
// Looping through the arrUserFirstNames array
arrUserFirstNames.forEach(userFirstName => {
console.log(userFirstName);
});
// Looping through the arrPrices array
arrPrices.forEach(price => {
console.log(price);
});
// looping through the arrCars array
arrCars.forEach(car => {
console.log(car);
});
In the above examples, the variables userFirstName, price and car are parameters that represent the current array item being processed. They are typically named in a single form of the array name. Another option is to use a generic name such as item or element.
The => operator is called a fat arrow symbol.
The general form of the .forEach() loop is as shown below.

With a .forEach() loop, you can perform any valid operation on the items in an array. For an array of numbers, for example, you could reduce each item by 10%.
// Changing numeric values with a loop
arrPrices.forEach(price => {
console.log(`Original price: ${price}`);
console.log(`Sale price (10% off): ${price - (price * 0.1)}`);
});
And for an array of strings, you could change all the characters in each item to lower case letters.
// Changing case of text characters in loop
arrUserFirstNames.forEach(userFirstName => {
console.log(`Original name: ${userFirstName}`);
console.log(`Lower case name: ${userFirstName.toLowerCase()}`);
});
Note that the .forEach() loop does not change the items in the array. It instead creates of a copy of the original array.
Creating an empty array
There is one situation where you might use let rather const when declaring an array. That is when you do not yet know what values will be stored in the array.
When you need to first create an empty array and populate it later with values, you could do the following:
// === Creating an empty array to populate later ===
// Create array without items
let arrLastNames = [];
// Populate empty array
arrLastNames = ["Bloggs", "Smith", "Murphy", "Higgins"];
console.log(`Array items: ${arrLastNames}`);
Array destructuring
Array destructuring allows you to extract items from an array and assign them to variables.
You could do this:
// Create and populate new array
const arrFruits = ["Apple", "Banana", "Orange", "Pear"];
// Assign array items to variables without destructuring
let fruit1 = arrFruits[0];
let fruit2 = arrFruits[1];
let fruit3 = arrFruits[2];
let fruit4 = arrFruits[3];
console.log(`Fruit 1: ${fruit1}`);
console.log(`Fruit 2: ${fruit2}`);
console.log(`Fruit 3: ${fruit3}`);
console.log(`Fruit 4: ${fruit4}`);
A more modern option is to use array destructuring. This enables array items to be ‘unpacked’ in a single line of code.
With array destructuring, your code can be simplified to:
// Assign array items to variables with destructuring
let [fruitOne, fruitTwo, fruitThree, fruitFour] = arrFruits;
console.log(`Fruit 1: ${fruitOne}`);
console.log(`Fruit 2: ${fruitTwo}`);
console.log(`Fruit 3: ${fruitThree}`);
console.log(`Fruit 4: ${fruitFour}`);
You can skip items in the array as follows:
// Create and populate new array
const arrCities = ["Dublin", "Cork", "Belfast", "Derry"];
// Skipping an array item while destructuring
let [firstCity, , thirdCity] = arrCities;
console.log(`Array item at position [0]: ${firstCity}`);
console.log(`Array item at position [2]: ${thirdCity}`);
Try it yourself
In your workfile...
---
Create an array called arrColours
with five different colour names. Then, in the console, output:
- The first colour
- The last colour using the array's length
- The total number of colours in the array
---
Create an array called arrMonths
with the first three months of the year. Then:
- Use destructuring to assign each month to a separate variable
- Try destructuring with skipping the second month
- Output the extracted values to the console
More learning resources
Tutorial Quiz
Tutorial Podcast
Sample AI prompts
Create a visual explanation of how array indexing works in JavaScript. Compare an array to a real-world example like numbered parking spots in a parking lot, or seats in a theatre. Explain why arrays start at index 0 instead of 1, and demonstrate common indexing mistakes beginners should avoid.
Write a step-by-step guide explaining how forEach
works with simple arrays of strings and numbers. Explain how forEach
accesses each item in the array.
Explain array destructuring using a real-world analogy, like unpacking a box of items. Show how destructuring compares to traditional array access methods, demonstrate skipping elements, and explain practical use cases where destructuring makes code more readable and efficient.