Learning Goals
At the end of this Tutorial, you will be able to:
- Access and manipulate HTML forms using JavaScript
- Handle form submission events and prevent default behavior
- Validate user input before form submission
- Extract and process form data
Download a new workfile on your computer as described at the link below.
About HTML forms
Forms are an essential part of web applications, allowing users to input data that can be processed by JavaScript and stored in a database.
Copy the following basic form structure into the HTML part of your forms.html workfile:
<!-- Email and password form -->
<h2>A basic form</h2>
<form id="userForm" onsubmit="processForm(event)">
<label for="userName">Name:</label>
<input type="text" id="userName" name="userName"><br><br>
<label for="userEmail">Email:</label>
<input type="email" id="userEmail" name="userEmail"><br><br>
<button type="submit">Submit</button>
</form>
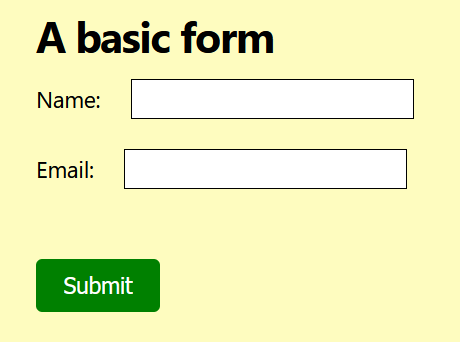
When working with forms in JavaScript, you will typically want to:
- Capture the form submission event (user clicking the Submit button)
- Validate user input
- Process form data
- Provide feedback to users
Accessing form data with JavaScript
The simplest way to access the details entered in HTML forms with JavaScript is with code such as that below. Copy this code to the JavaScript part of your forms.html workfile.
// Function to process the form
function processForm(event) {
event.preventDefault(); // prevents the web page reloadiing
// Get values entered by the user
const userNameInput = document.getElementById('userName').value;
const userEmailInput = document.getElementById('userEmail').value;
// Output the values to the console
console.log(`Name entered by user: ${userNameInput}`);
console.log(`Email entered by user: ${userEmailInput}`);
}
Enter some details in the form, click Submit, and verify your form runs correctly.
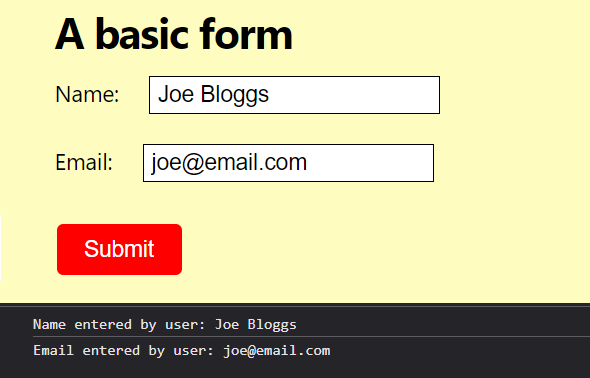
About event.preventDefault()
By default, clicking the form Submit button will trigger two actions:
- The data in the form will be submitted.
- The web page will reload.
Typically, you will want to validate the entered data before the form is submitted. Also, you will not want the web page to reload in the browser.
Form validation
Validation ensures that user input meets your requirements before processing. JavaScript can perform validation when the form is submitted.
The type="email" form input field has built-in web browser validation that checks for a correct email address format.
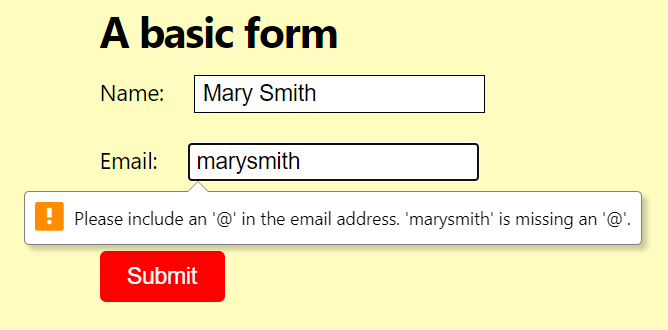
You can add validation to other input fields JavaScript. For example, add the code below to your processForm() function.
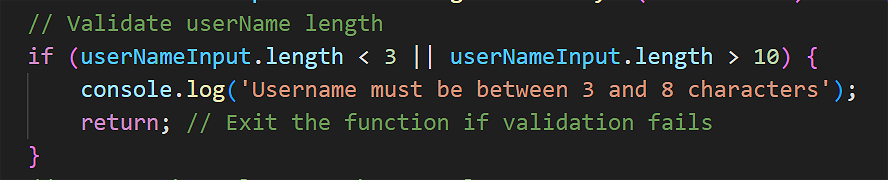
After form has been successfully submitted, you can use the reset() method to clear the form.
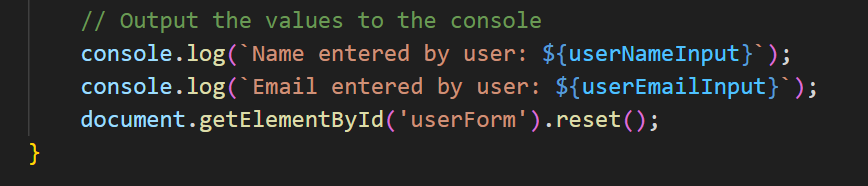
More learning resources
Tutorial Quiz
Tutorial Podcast
Sample AI prompts
In an HTML file, create a simple registration form with the following fields:
- Username (text input)
- Email (email input)
- Password (password input)
- Confirm Password (password input)
In the same file, create a plain JavaScript code:
- Validate that all fields are filled out
- Check that passwords match
- Output the form data to the console
- Use an event handler instead of an event listener
- user innerText instead of textContent
In the <head> of the HTML file, add two CSS classes to style a DIV tag with an id of "message" depending on whether the form passes validation or not.
After the form is submitted, use JavaScript to create the "message" DIV tag, assign the appropriate CSS class to it with classList.add(), and append it to the form.
Finally, reset the form.