Learning Goals
At the end of this Tutorial, you will be able to:
- Use the <script> tag to include JavaScript code in an HTML file and to link to external JavaScript files.
- Ensure that JavaScript code is non-blocking — it does not delay loading of HTML content.
- Ensure that JavaScript does not attempt to access the HTML content until all HTML elements are loaded.
- Open and use the JavaScript Console in your web browser’s DevTools.
Create a new folder on your computer named javascript and inside it create a sub-folder named exercises.
Download a new workfile on your computer as described at the link below.
📄 Adding JavaScript: Exercises
Also, download this empty JavaScript file named script.js to your javascript/exercises folder.
JavaScript and the <script>
tag
The two most efficient ways to add JavaScript to a web page are as shown below:
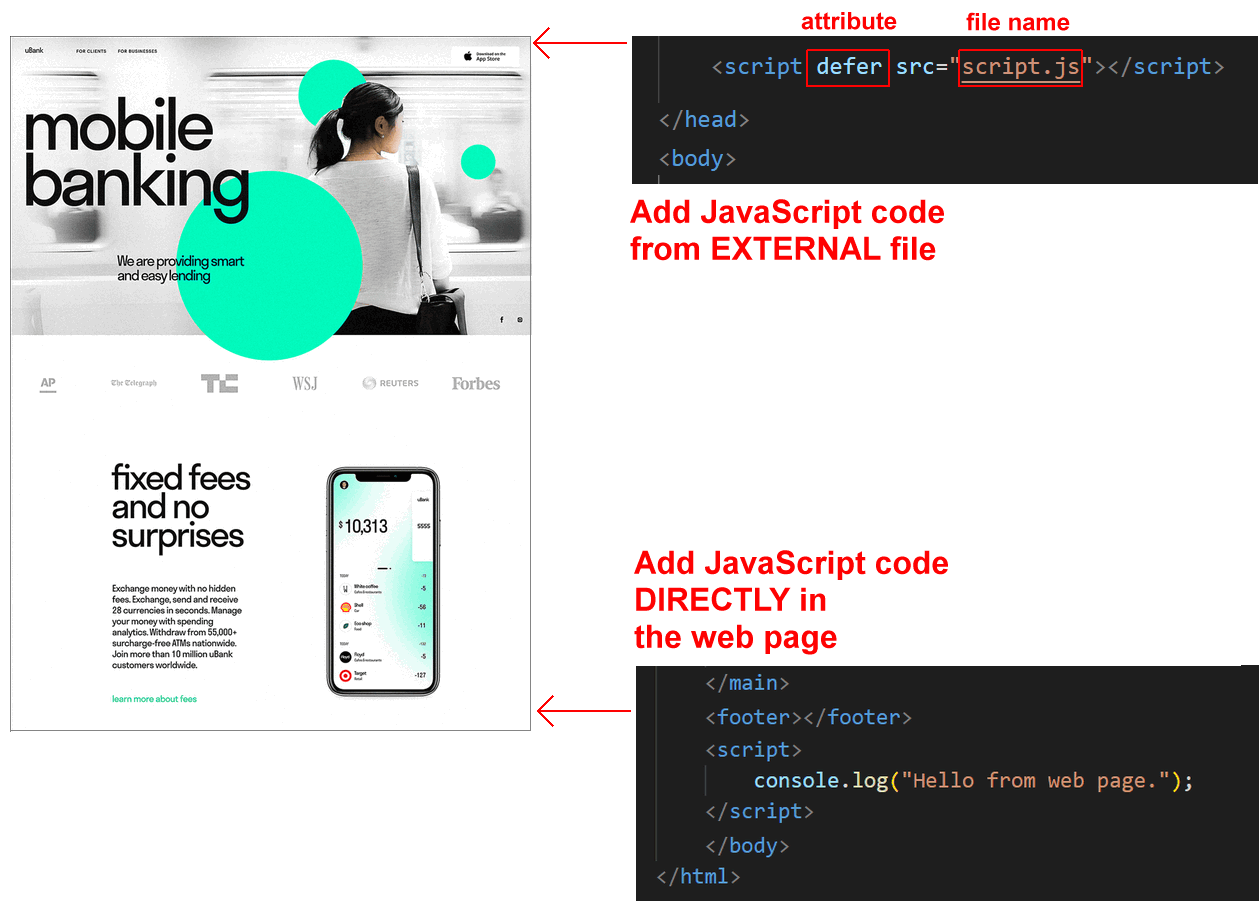
Both methods are:
- Non-blocking: Users experience faster page load time because the JS code does not delay the rendering of the HTML. And you should see fewer audit messages like this on Google"s PageSpeed Insights site.
- Access to HTML elements: The JS code will try to access elements in the HTML file only after the file is loaded. So you should not see errors like this in the JavaScript Console.
External JavaScript files
Here are the benefits of using external JavaScript files:
- They separate the JavaScript from the HTML code.
- They make the HTML and JavaScript easier to read and maintain
- On multi-page websites, cached JavaScript files can speed up page loads.
Do not include <script> tags in external JavaScript files.
Working with your web browser DevTools
Your web browser’s Developer Tools (also known as DevTools) includes a JavaScript Console. To display this:
- Windows (all browsers): Press Ctrl + Shift + i
- Mac (Safari browser): Press ⌘ + ⌥ + i Alternatively, right-click on the webpage and choose Inspect Element from the context menu.
Changing the DevTools position
To position or ‘dock’ the DevTools window:
- Open the DevTools window.
- Near the top-right of the DevTools window, click the vertical ellipses (...) icon.
- Click the docking position you want for the DevTools window.
Changing the DevTools font size
To change the font size in the DevTools window, select the window, and then press the Ctrl key followed by the plus (+) or minus (-) key. To reset, press Ctrl and 0.
Working with the JavaScript Console
Of the various tabs shown across the top of DevTools window, click Console to display the JavaScript Console.
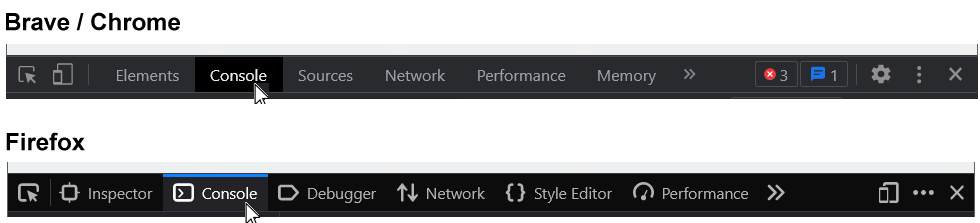
You can use the Console to display or ‘log’ information as part of your JavaScript coding process.
- In your code, you can use console.log() statements to inspect the values of variables and properties of objects.
- Any coding errors will appear in the Console, along with the line number and a short explanatory message.
In summary, the JavaScript Console provides a working space for you to try out and debug JavaScript code in real-time.
Adding JavaScript to HTML of a web page
Follow these steps:
- Open the adding-javascript.html web page. Just before the closing </body> tag, enter the following JavaScript code snippet:
The bottom of your web page should now look as follows:<script> console.log("Hello from web page."); </script>
- Save and reload the web page. You should see the following message in your browser's JavaScript Console:
✅ You have now added some JavaScript code directly to the HTML of a web page.
For practice, add some more console.log() statements to your adding-javascript.html workfile.
Adding an external JavaScript file to a web page
Follow these steps:
- In your adding-javascript.html workfile, add the following line just before the closing </head> tag.
Your web page should now look as follows:<script defer src="script.js"></script>
- Open your script.js file and add the following new line:
Your JavaScript file should now look as follows.console.log("Hello from external JavaScript file.");
- Save the JavaScript file and then reload your web page. You should see the following message in the JavaScript Console:
✅ You have now linked your web page to an external JavaScript file.
For practice, add some more console.log() statements to your script.js workfile.
The ;
statement terminator
In JavaScript, it is a good idea to end your statements with a semi-colon character ;
This is not strictly necessary, but it helps to make your code more readable.
JavaScript and AI tools
Here are some AI tools to help you on your JavaScript learning journey:
- GitHub Copilot (Extension to VS Code. Free version available.)
- Microsoft Copilot (Similar to free version of ChatGPT.)
- ChatGPT (Free version available.)
- Google Gemini (Free version available.)
- Claude (Free version available ✅ Highly recommended.)
More learning resources
Tutorial Quiz
Tutorial Podcast
Sample AI prompts
I want to add some JavaScript code directly in my HTML page. Can you explain how to use the <script>
tag and where to place it in my HTML file for the best performance?
Why should I use the defer
attribute when loading an external JavaScript file into a web page? How does it make my webpage load faster?
Can you help me write a simple script that displays "Hello, world!" in the JavaScript Console of DevTools when I load my webpage?
Why is it important that JavaScript code should be the last item to be added to a web page after all the other content, such as text and images, have already loaded in the web browser?
What are the pros and cons of putting JavaScript in external files versus directly in HTML? When should I use each approach?